Programming
We are in the era of fast-pace advancing technology and machines. To interact with machines, we program them. To understand programming, think of a machine as a child who doesn’t know what to do but when instructed, does what was supposed to do. This can be understood as Programming i.e. Programming is the process of giving instructions to a machine (Computer) to carry out intended operations and functionalities.
A computer program is a code that is fed as instructions to the Computer. The code is written by the programmers in a specific syntactical form known as a programming language. Computers do not understand the text-based instructions (High-level language), so, they are needed to be translated to the machine-readable form which is known as machine code (Low-level language). Machine code is in the form of binary i.e. 0s and 1s.
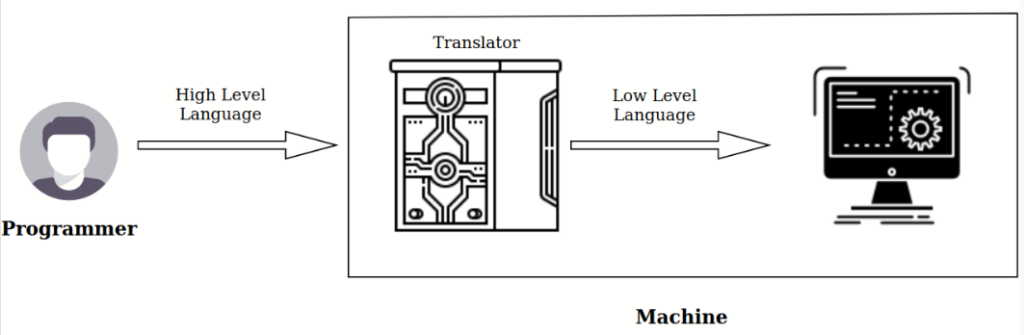
Languages are classified into:
• Low Level Language
• High Level Language
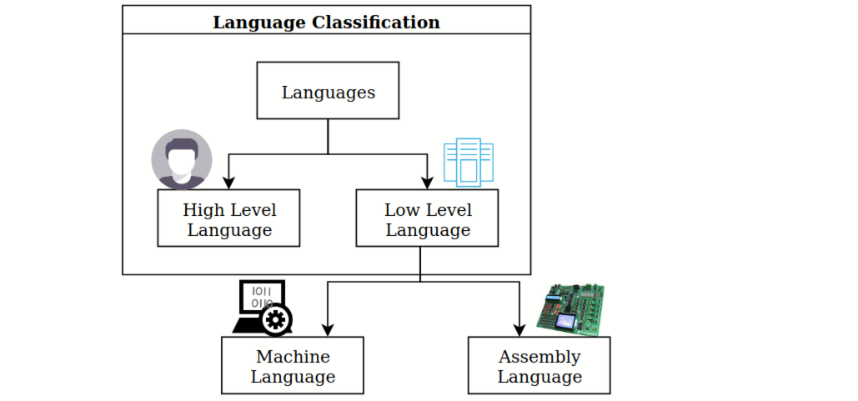
The high-level language allows a programmer to write programs that are independent of a particular type of computer and are text-based. The high-level languages are considered as high-level because they are closer to human languages than machine-level languages. Eg: C, C++, Python, Java, etc.
Eg: Python program to print sum of two numbers
a=900
b=850
c=a+b
print(“The Sum of a and b: “, c)
Machine language is the low-level language that is most difficult for a human to understand and work with. Machine language uses 0s and 1s. Digital devices recognize everything in binary irrespective of data format i.e. every type of data is understood as 0s and 1s whether it is a text, video, audio, or any other command.
Eg: “Hello World” is understood as 01001000 01100101 01101100 01101100 01101111 00100000 01010111 01101111 01110010 01101100 01100100 00001010
Assembly language is a compiled, low-level language for some microprocessors and programmable devices and it directly works with the internal structure of CPU. It lies somewhere between High-Level Language and Machine language. Assembly language program consists of mnemonics that are translated into machine code.
Eg: Addition of 8-bit numbers on 8051 microcontroller
MOV R0,#20H; set source address 20H to R0
MOV R1,#30H; set destination address 30H to R1
MOV A,@R0; take the value from source to register A
MOV R5,A; Move the value from A to R5
MOV R4,#00H; Clear register R4 to store carry
INCR 0; Point to the next location
MOV A,@R0; take the value from source to register A
ADD A,R5; Add R5 with A and store to register A
JNC SAVE
INC R4; Increment R4 to get carry
MOV B,R4; Get carry to register B
MOV @R1,B; Store the carry first
INCR 1; Increase R1 to point to the next address
SAVE: MOV @R1,A; Store the result
HALT: SJMP HALT ; Stop the program
Programming is “giving instructions or commands to a machine to get some task done by it.”